Java 8 New Features With Example
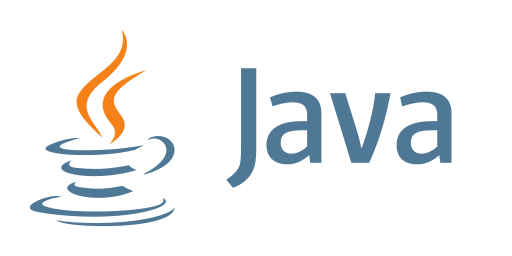
Java 8 was a major release of the Java programming language that brought several new features and enhancements. Below are some of the most notable features along with code examples to illustrate their usage:
- Lambda Expressions: Lambda expressions are a new way to write functional interfaces in Java. A functional interface is an interface with a single abstract method. Here's an example of a functional interface and its implementation using a lambda expression:
1interface MathOperation {
2 int operation(int a, int b);
3}
4
5MathOperation addition = (int a, int b) -> a + b;
6System.out.println(addition.operation(1, 2)); // Output: 3
7
- Method references: Method references provide a way to refer to methods by their names. Here's an example of using a method reference to print all the elements of a list:
1List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
2numbers.forEach(System.out::println);
3
- Stream API: The Stream API is a new feature that allows you to perform functional-style operations on collections of elements. Here's an example of using the Stream API to filter a list of numbers and print the square of each number that is greater than 3:
1List<Integer> numbers = Arrays.asList(1, 2, 3, 4, 5);
2numbers.stream()
3 .filter(n -> n > 3)
4 .map(n -> n * n)
5 .forEach(System.out::println);
6
- Default Methods: Java 8 introduces default methods in interface, it allows the developer to add new methods to the interfaces without breaking the existing implementation of these interfaces. Here is an example of a default method in an interface
1interface MyInterface {
2 void existingMethod();
3 default void newMethod() {
4 // Default implementation
5 }
6}
7
8class MyClass implements MyInterface {
9 public void existingMethod() {
10 // Implementation
11 }
12}
13
- Date and Time API: Java 8 introduced a new Date and Time API (JSR 310) that replaces the old and outdated java.util.Date and java.util.Calendar classes. The new API is immutable, thread-safe, and much more powerful than the old one. Here are some code examples that show how to use the new Date and Time API:
Creating a LocalDate :
1LocalDate date = LocalDate.of(2022, Month.JANUARY, 25);
2System.out.println(date); // Output: 2022-01-25
In this example, we create a new LocalDate object that represents the date January 25, 2022. The LocalDate class only represents a date without a time or time zone.
Creating a LocalTime:
1LocalTime time = LocalTime.of(13, 45, 20);
2System.out.println(time); // Output: 13:45:20
3
In this example, we create a new LocalTime object that represents the time 1:45:20 PM. The LocalTime class only represents a time without a date or time zone.
Creating a LocalDateTime:
1LocalDateTime dateTime = LocalDateTime.of(2022, Month.JANUARY, 25, 13, 45, 20);
2System.out.println(dateTime); // Output: 2022-01-25T13:45:20
3
In this example, we create a new LocalDateTime object that represents the date and time January 25, 2022 1:45:20 PM. The LocalDateTime class represents both a date and a time, but does not contain any information about the time zone.
Creating a ZonedDateTime:
1ZonedDateTime zonedDateTime = ZonedDateTime.of(2022, Month.JANUARY, 25, 13, 45, 20, 0, ZoneId.of("Europe/Paris"));
2System.out.println(zonedDateTime); // Output: 2022-01-25T13:45:20+01:00[Europe/Paris]
3
In this example, we create a new ZonedDateTime object that represents the date, time and time zone January 25, 2022 1:45:20 PM in Europe/Paris. The ZonedDateTime class contains all the information from LocalDateTime and also the time zone information.
These are just a few examples of how to use the new Date and Time API in Java 8. There are many other classes and methods in the API that allow you to perform various operations on dates and times, such as parsing and formatting, arithmetic, and time zone conversions.
- Optional Class: Java 8 introduced a new class called Optional, it is used to represent a value that may or may not be present. Here's an example of how to use the Optional class to avoid null pointer exceptions:
1Optional<String> optional = Optional.of("value");
2System.out.println(optional.isPresent()); // Output: true
3System.out.println(optional.get()); // Output: value
4
- Nashorn JavaScript Engine: Java 8 introduced a new JavaScript engine called Nashorn, which replaces the older Rhino engine. Here's an example of how to use Nashorn to evaluate a JavaScript expression:
1import javax.script.ScriptEngine;
2import javax.script.ScriptEngineManager;
3import javax.script.ScriptException;
4
5public class NashornExample {
6 public static void main(String[] args) {
7 ScriptEngine engine = new ScriptEngineManager().getEngineByName("nashorn");
8 try {
9 Object result = engine.eval("2 + 3");
10 System.out.println(result); // Output: 5
11 } catch (ScriptException e) {
12 e.printStackTrace();
13 }
14 }
15}
16
In this example, we first create a new ScriptEngineManager object, which is used to create instances of the Nashorn engine. Then, we use the getEngineByName() method to get an instance of the Nashorn engine. Next, we use the eval() method of the engine to evaluate the JavaScript expression "2 + 3", which returns the result 5.
You can also use the Nashorn engine to evaluate more complex JavaScript code, such as functions, variables and objects. Here's an example of how to define a JavaScript function and call it from Java:
1ScriptEngine engine = new ScriptEngineManager().getEngineByName("nashorn");
2engine.eval("function greet(name) { return 'Hello, ' + name; }");
3Invocable invocable = (Invocable) engine;
4Object result = invocable.invokeFunction("greet", "John");
5System.out.println(result); // Output: Hello, John
6
In this example, we first define a JavaScript function called "greet" using the eval() method. Then, we cast the engine object to an Invocable object, which allows us to invoke the function. Finally, we use the invokeFunction() method to call the greet function with the argument "John" and print the result.
- Parallel Array Sorting: Java 8 introduced a parallel sorting algorithm for arrays which can sort large arrays of data faster