Java 12 New Features With Example
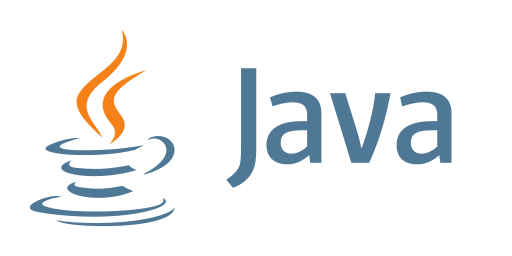
Switch Expressions: It allows using the switch statement as an expression to return a value. It introduces a new arrow operator -> to separate the case label from the expression. Example:
1int numLetters = switch (day) {
2 case MONDAY, FRIDAY, SUNDAY -> 6;
3 case TUESDAY -> 7;
4 case THURSDAY, SATURDAY -> 8;
5 case WEDNESDAY -> 9;
6}
Text Blocks: It allows for multiline string literals, which makes it easier to write strings that contain multiple lines of text. The string is enclosed in triple quotes (""") and the whitespace is preserved. Example:
1String html = """
2<html>
3 <body>
4 <p>Hello, World!</p>
5 </body>
6</html>""";
7
JVM Constants API: It provides a way to access the constant pool of a class file in the JVM. The new class jdk.internal.vm.constants.ConstantPool provides methods to access the constants of a class file. Example:
1ConstantPool cp = ClassFile.read(className).constantPool();
2String className = cp.getClassAt(cp.getSize() - 1).getName();
3
Improved Startup Time: It has improved the startup time by reducing the number of classes and resources that are loaded during startup.
Microbenchmark Suite: It has added a new JMH-based microbenchmark suite, which is a set of tools for writing and running microbenchmarks in the JDK.
Deprecation of the Nashorn JavaScript Engine: The Nashorn JavaScript engine, which was first introduced in Java 8, has been deprecated and will be removed in a future release.
Other improvements:
- Improved handling of Unicode 11.0
- Better C++ and C99 support
- Enhanced support for ZGC (Z Garbage Collector)
These are some of the main new features and improvements included in Java 12, but it also includes other changes to existing features and bug fixes. It's worth noting that some of these features are experimental, and their behavior may change in future versions of Java.