Java 11 New Features With Example
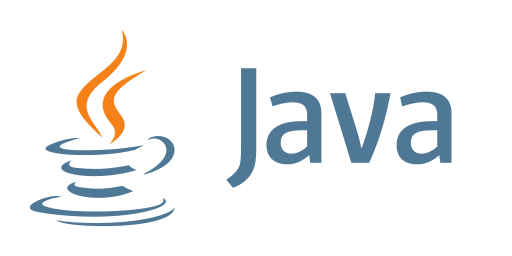
1. Local-Variable Syntax for Lambda Parameters: Java 11 introduced a new way to declare the parameter types of a lambda expression, called "local-variable syntax for lambda parameters". This allows the type of a lambda parameter to be inferred from the context, making the code more concise and readable. Here is an example:
1List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
2
3// Prior to Java 11, the type of the lambda parameters had to be explicitly declared
4 names.sort((String a, String b) -> a.compareTo(b));
5
6// Java 11 allows the type to be inferred from the context
7 names.sort((a, b) -> a.compareTo(b));
As you can see in the example above, the type of the lambda parameters, a
and b
are inferred from the context, they are string type, this is the same as explicitly declaring them as String a
, String b
which was mandatory before Java 11. This feature makes the code more readable, and less verbose.
It's important to note that local-variable syntax for lambda parameters is not limited to just the type, the variable name can also be inferred from the context.
1List<String> names = Arrays.asList("Alice", "Bob", "Charlie");
2
3// Prior to Java 11, the variable name of lambda parameters had to be explicitly declared
4names.forEach(name -> System.out.println(name));
5
6// Java 11 allows the variable name to be inferred from the context
7names.forEach(System.out::println);
In this example, the variable name
is inferred from the context, and the forEach method will print each element of the list. This is the same as explicitly declaring the variable name
and using it in the lambda expression.
2. HTTP/2 Client: Java 11 includes a new HTTP/2 client that provides a more efficient way to handle HTTP requests and responses. This is an example of how to use the new client:
1HttpClient client = HttpClient.newBuilder().build();
2HttpRequest request = HttpRequest.newBuilder().uri(new URI("https://www.example.com")).build();
3HttpResponse<String> response = client.send(request, HttpResponse.BodyHandlers.ofString());
4System.out.println(response.body());
3. Flight Recorder: Java 11 includes a new feature called Flight Recorder, which allows you to collect detailed performance data about your Java application at runtime. This can be useful for troubleshooting performance issues and identifying bottlenecks in your code. Here is an example of how to use Flight Recorder:
1// Enable Flight Recorder
2-XX:+FlightRecorder
3
4// Start recording
5jcmd <pid> JFR.start
6
7// Stop recording
8jcmd <pid> JFR.stop
9
10// Dump the recording to a file
11jcmd <pid> JFR.dump filename=recording.jfr
4. Unicode 10 Support: Java 11 added support for Unicode 10, which includes several new characters and emojis. Here is an example of how to use these new characters in a Java program:
1String s = "This is a string with a 🦊 (fox) and a 🦄 (unicorn).";
2System.out.println(s);
Java 11 also includes new Unicode properties and scripts, which are used to help identify the type of character and improve text processing. For example, the script "Emoji" has been added to identify all the emoji characters.
Example:
1Character.UnicodeScript script = Character.UnicodeScript.of('🦊');
2System.out.println(script); //output Emoji
5. Nest-Based Access Control: Java 11 introduced a new feature called Nest-based access control, which allows inner classes to access private members of their outer classes more efficiently.
1class Outer {
2 private int x;
3 class Inner {
4 int getX() {
5 return x;
6 }
7 }
8}
6. Epsilon GC: Java 11 introduced a new garbage collector called Epsilon GC, which does not perform any heap memory reclamation. Instead, it simply allocates memory as needed and throws an OutOfMemoryError when the heap runs out of memory. This can be useful in certain situations, such as when you need to perform a one-off task that is not memory-bound, or when you need to test the maximum heap size of a system.
Here's an example of how to use Epsilon GC in Java:
1-XX:+UnlockExperimentalVMOptions -XX:+UseEpsilonGC
This command line flag allows to enable the Epsilon GC, in this GC all the memory allocation is done in the heap and when the heap is full and can't allocate more memory, an OutOfMemoryError is thrown.
It's important to note that Epsilon GC is not a general-purpose garbage collector, it's recommended to use it in specific use cases such as testing, high performance short-lived applications where the heap size is known, or when the heap size is not critical and the system is expected to run out of memory.
Additionally, Epsilon GC is experimental and not recommended to be used in production environments, as it may cause applications to crash due to lack of memory.
These are just a few examples of the new features and improvements that were introduced in Java 11. Other new features include the removal of the Java EE and CORBA modules.